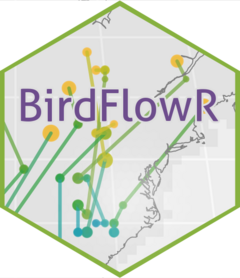
Are locations and distributions covered by the model for the given time
Source:R/is_location_valid.R
is_location_valid.Rd
A location or and time is valid if the model has a non zero probability of movement into and out of that location at that time. An invalid state either has zero probability of movement into and out of it, or isn't covered by the model at all. Similarly distributions are valid if all of the probability is in cells for which movement is modeled.
Usage
is_location_valid(bf, i, x, y, timestep, date)
is_distr_valid(bf, distr, timestep, date, return_mask = FALSE)
Arguments
- bf
A BirdFlow object.
- i
State space index (location).
- x
X coordinates in the
bf
's CRS (crs(bf)).- y
T coordinate.
- timestep
The timestep.
- date
Date in any format accepted by
lookup_timestep()
.- distr
One or more distributions in vector or matrix form representing a probability for each active cell in the model.
- return_mask
If TRUE return a mask with the same dimensions as
distr
which is TRUE for all cells that can have valid non-zero values. This is conditioned on the timestep associated with each distribution.
Value
A logical vector, 'TRUEif valid
FALSEotherwise with values for each input location or distribution. See
return_mask` for an exception.
Details
For is_location_valid()
there are 2 ways of inputting locations. Only
one should be used: either i
a vector of location indices
(see xy_to_i()
), or x
and y
.
For both functions time should be input either as timestep
or date
.
The number of timesteps or dates should either be 1 or match to the number of locations or distributions; if singular it will be applied to all.
A location is invalid if any of the following apply:
The location isn't in the model extent.
The location doesn't correspond to an active cell in the model; it's masked out by the static mask.
The location isn't a valid state at the given timestep or date; it's excluded by the dynamic mask or by state or other sparsification see
sparsify()
.The timestep isn't valid, or the date doesn't have an associated timestep. The second applies only to BirdFlow models that don't cover the whole year.
A distribution is invalid for similar reasons but applied to all the locations that have non-zero values.
Examples
bf <- BirdFlowModels::amewoo
timestep <- 3
# Sample two valid locations from a distribution
distr <- get_distr(bf, timestep, from_marginals = TRUE)
locs <- sample_distr(distr, n = 2)
i <- apply(locs, 2, function(x) which(as.logical(x)))
is_location_valid(bf, i, timestep = timestep)
#> [1] TRUE TRUE
# Sample a few invalid locations
i <- sample(which(distr == 0), 2)
is_location_valid(bf, i, timestep = timestep)
#> [1] FALSE FALSE